Berlin Clock
The Berlin Clock, also known as the 'Mengenlehreuhr,' displays time with a series of coloured flashing lights.
This clock works in base 5: www.3quarks.com/en/BerlinClock/index.html
I printed an acrylic board for the lights, which were WS2812 (aka Neopixel strips).
At first I wanted to solder individual lights, to fit to the acrylic board.
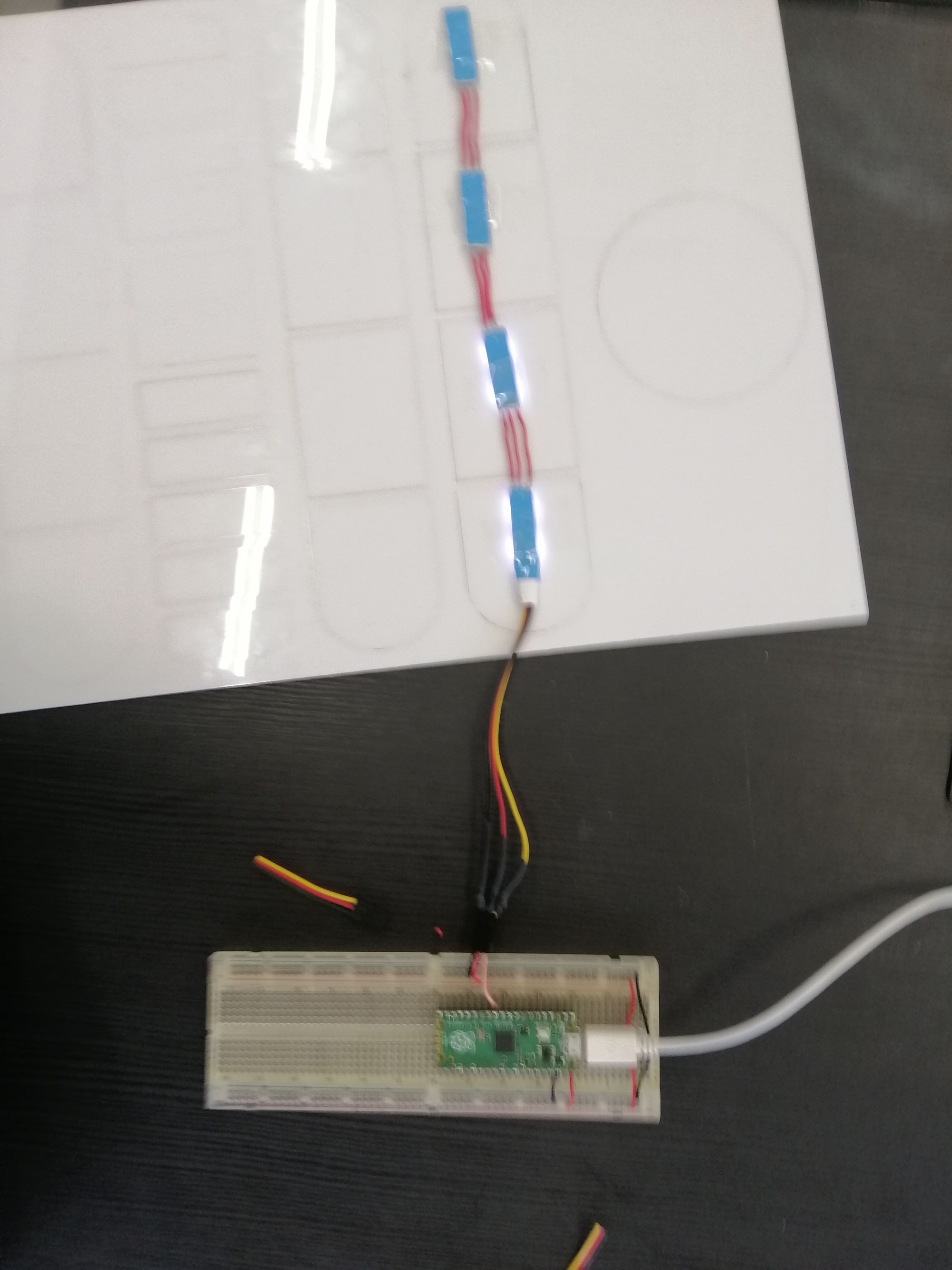
However, this was time-consuming so I switched to using standard size lights and scale the board to the standard size. When I was done, I wired up all 4 rows of lights and the LED circle to the acrylic board.
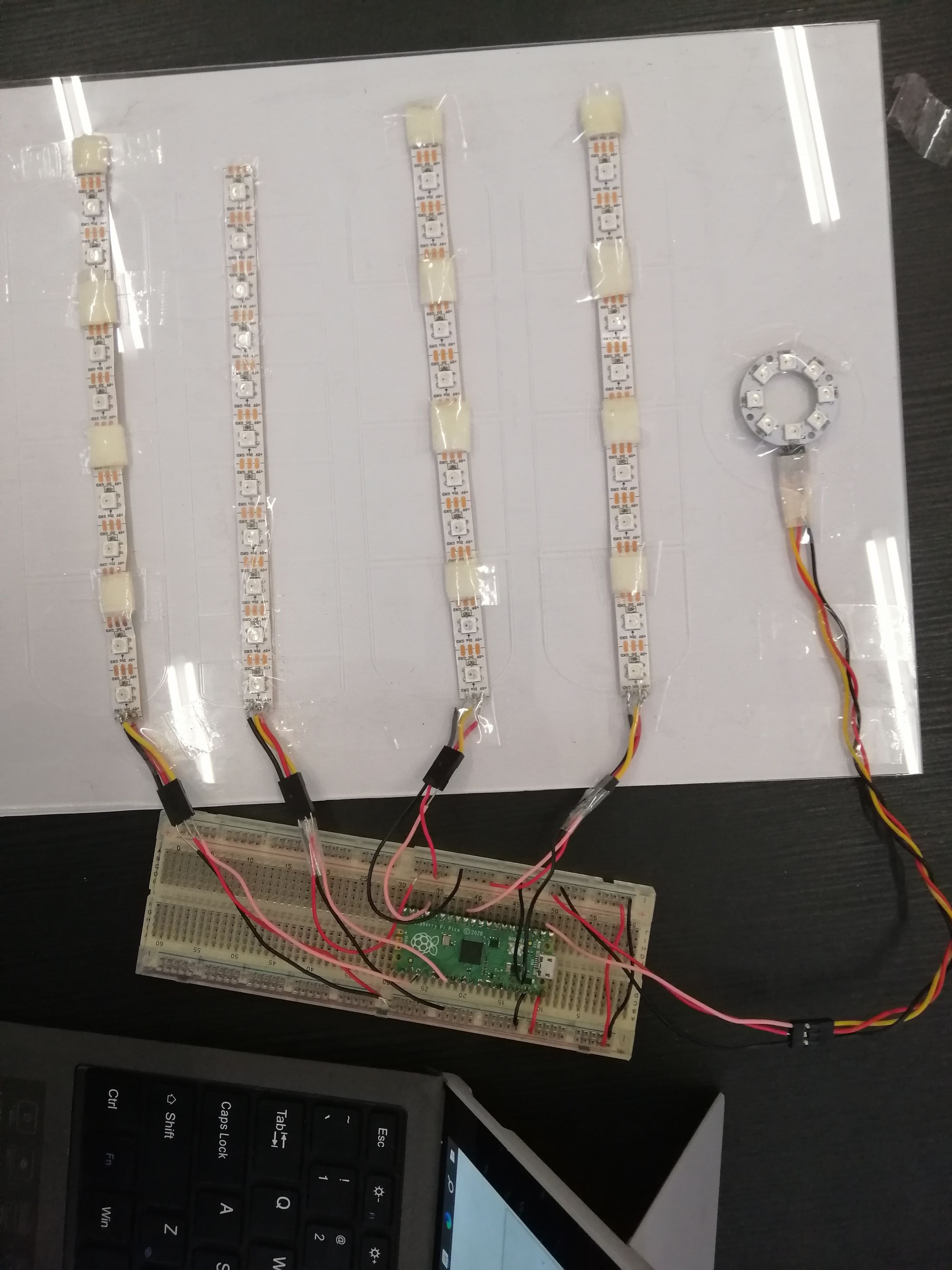
The Neopixel strips were coded:
#import
import time
from neopixel import Neopixel
rtc = machine.RTC()
YELLOW = (255, 255, 0)
RED = (0, 255, 0)
BLACK = (0, 0, 0)
secSMID = 0
minSMID = 1
hrSMID = 2
hrRow1NumBlocks = 4 #constant
hrRow1NumLightsInOneBlock = 3 #change this according to number of lights in one block
hrRow1NumLightsInAll = hrRow1NumLightsInOneBlock * hrRow1NumBlocks
hrRow2NumBlocks = 4 #constant
hrRow2NumLightsInOneBlock = 3 #change this according to number of lights in one block
hrRow2NumLightsInAll = hrRow2NumLightsInOneBlock * hrRow2NumBlocks
minRow1NumBlocks = 11 #constant
minRow1NumLightsInOneBlock = 1 #change this according to number of lights in one block
minRow1NumLightsInAll = minRow1NumLightsInOneBlock * minRow1NumBlocks
minRow2NumBlocks = 4 #constant
minRow2NumLightsInOneBlock = 3 #change this according to number of lights in one block
minRow2NumLightsInAll = minRow2NumLightsInOneBlock * minRow2NumBlocks
secRowNumLightsInAll = 8 #change this according to number of lights
#seconds of current time
def show_second(secs):
pixelssec = Neopixel(secRowNumLightsInAll, secSMID, 1, "GRB")
pixelssec.brightness(50)
if secs % 2 == 1:
pixelssec.set_pixel_line(0, secRowNumLightsInAll - 1, YELLOW)
else:
pixelssec.set_pixel_line(0, secRowNumLightsInAll - 1, BLACK)
pixelssec.show()
#the hour of current time (needs to be in same loop because they share 1 state machine)
def show_hour(hr):
#first hours row
hrRow1NumBlocksLit = hr // 5
hrRow1NumLightsLit = hrRow1NumBlocksLit * hrRow1NumLightsInOneBlock
pixelshr1 = Neopixel(hrRow1NumLightsInAll, hrSMID, 9, "GRB")
pixelshr1.brightness(40)
#all the blocks that need to be lit
for iBlock in range(hrRow1NumBlocksLit):
for iLight in range(
hrRow1NumLightsInOneBlock): #for each light in the block:
if iLight < hrRow1NumLightsInOneBlock:
pixelshr1.set_pixel(iLight, RED) #make it red
else: #the last light of each block is not used
pixelshr1.set_pixel(iLight, BLACK) #make it black
#the rest of the blocks
pixelshr1.set_pixel_line(hrRow1NumLightsLit, hrRow1NumLightsInAll - 1,
BLACK)
pixelshr1.show()
#second hours row
hrRow2NumBlocksLit = hr % 5
hrRow2NumLightsLit = hrRow2NumBlocksLit * hrRow2NumLightsInOneBlock
pixelshr2 = Neopixel(hrRow2NumLightsInAll, hrSMID, 13, "GRB")
pixelshr2.brightness(40)
#all the blocks that need to be lit
for iBlock in range(hrRow2NumBlocksLit):
for iLight in range(
hrRow2NumLightsInOneBlock): #for each light in the block:
if iLight < hrRow2NumLightsInOneBlock:
pixelshr2.set_pixel(iLight, RED) #make it red
else: #the last light of each block is not used
pixelshr2.set_pixel(iLight, BLACK) #make it black
#the rest of the blocks
pixelshr2.set_pixel_line(hrRow2NumLightsLit, hrRow2NumLightsInAll - 1,
BLACK)
pixelshr2.show()
#the minutes of current time
def show_minute(mins):
#first min row
minRow1NumBlocksLit = mins // 5
minRow1NumLightsLit = minRow1NumBlocksLit * minRow1NumLightsInOneBlock
pixelsmin1 = Neopixel(minRow1NumLightsInAll, minSMID, 18, "GRB")
pixelsmin1.brightness(40)
for i in range(minRow1NumBlocksLit):
firstLight = i * minRow1NumLightsInOneBlock
lastLight = firstLight + minRow1NumLightsInOneBlock - 1
#if block number is divisible by 3 (15, 30, 45 mins)
if (i + 1) % 3 == 0:
pixelsmin1.set_pixel_line(firstLight, lastLight, RED) #make it red
else:
pixelsmin1.set_pixel_line(firstLight, lastLight,
YELLOW) #make it yellow
pixelsmin1.set_pixel_line(minRow1NumLightsLit, minRow1NumLightsInAll - 1,
BLACK)
pixelsmin1.show()
#second hours row
minRow2NumBlocksLit = mins % 5
minRow2NumLightsLit = minRow2NumBlocksLit * minRow2NumLightsInOneBlock
pixelsmin2 = Neopixel(minRow2NumLightsInAll, minSMID, 13, "GRB")
pixelsmin2.brightness(40)
#all the blocks that need to be lit
for iBlock in range(minRow2NumBlocksLit):
for iLight in range(
minRow2NumLightsInOneBlock): #for each light in the block:
if iLight < minRow2NumLightsInOneBlock:
pixelsmin2.set_pixel(iLight, YELLOW) #make it yellow
else: #the last light of each block is not used
pixelsmin2.set_pixel(iLight, BLACK) #make it black
#the rest of the blocks
pixelsmin2.set_pixel_line(minRow2NumLightsLit, minRow2NumLightsInAll - 1,
BLACK)
pixelsmin2.show()
while True:
timestamp = rtc.datetime()
_, _, _, _, hrs, mins, secs, _ = timestamp
show_second(secs)
show_hour(hrs)
show_minute(mins)
print(f"The time now is {hrs}:{mins}:{secs}")
time.sleep(0.99)
And after about 2 months of grueling wiring, lighting, and coding:
0:00
/